In this article, we’re going to cover what useEffect uses for, how to better understand it and use it in your react application
First, lets understand about:
What is pure function?
A pure function works as an independent function that returns the same output if we use the same input parameters, and it’s predictable.
React assumes that every component you write is a pure function, and returns the same JSX given the same inputs
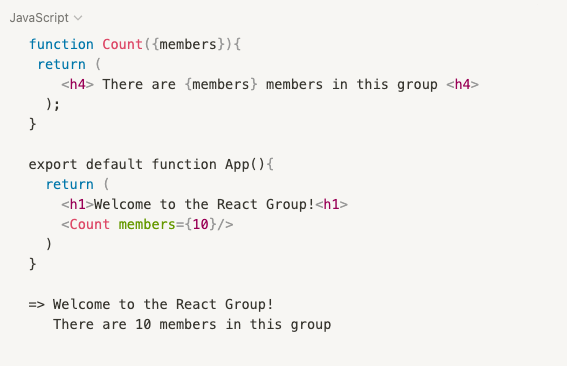
what is impure function?
An impure function is a function that modifies anything outside its body (like global variables). It mutates data outside of its lexical scope, and does not give us a predictable result.
what is side effect?
Side effect is a general concept about behaviors of functions that make data’s changed or is to mention impure functions.
In React, A side-effect can be any effect(programming logic) that is responsible for changing the state of your component. If the state changes, the component re-renders. we perform a side effect when we need to reach outside of our React to do something, but we have to do it on the side Not During Rendering
=> We should isolate side effect from rendering; therefore, useEffect here to help.
What is useEffect?
The useEffect
hook in React is a powerful tool for managing side effects in your components. You can use it to perform tasks like setting up a subscription, updating the state, or making an API request.
Common Side Effects in React
- Event: ADD/ remove event listener
- Observer pattern: subscribe/ unsubscribe
- Closures
- Timers: interval / setTimeOut / clearInterval / clearTimeOut
- useState
- Mounted / unmounted
- === strict equality comparison operator
- Call api
useEffect Syntax:
useEffect receives 2 parameters:
- callback function => required
- [] an array that contains the dependencies => not required
There are 3 cases of the useEffect:
- useEffect(callback)
- callback is called every time component re-render
- callback is called after component adding elements in DOM
- useEffect(callback,[])
- callback is called one time after component mounted
- useEffect(callback,[deps])
- callback is called every time deps are changed. If we add a deps(a variable) in [], callback is only called if that dep changed. If other things are changed callback will not act
Note: Common for all cases
- Callback is called after component is mounted
- Cleanup function is always called before component unmounted( use this to prevent leak memory when we work with window.addEventListener)
- cleanup function is always called before callback is called except the 1st mounted
The correct way to use the useEffect hook:
- Import the
useEffect
hook from thereact
library at the top of your component file. - Define the effect inside a call to
useEffect
, which takes a function that contains your effect logic. - Make sure to return a cleanup function from your effect if necessary(not required in every case). This function will be run when the component is unmounted or the effect is re-run.
- Pass an array of dependencies as the second argument to
useEffect
. This array should include any values from the component’s state or props that the effect depends on. - When the values in the dependency array change, the effect will be re-run. If the array is empty, the effect will only run once, when the component is first mounted.
Now lets do an example for each case of the cases that we just mentioned above
Case 1:
In this example, the useEffect is being used to update DOM
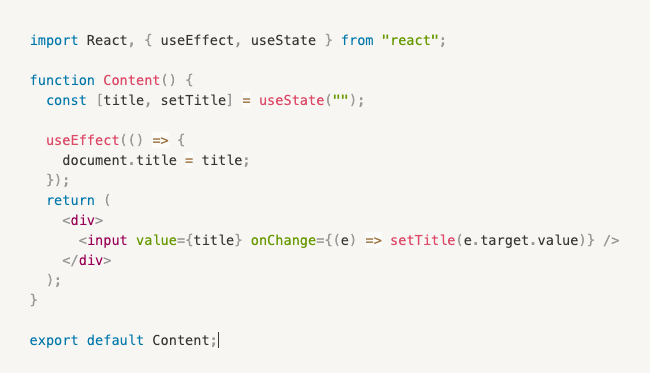
Case 2:
- In this example, the useEffect is being to make a request to an API and update the state with the response.
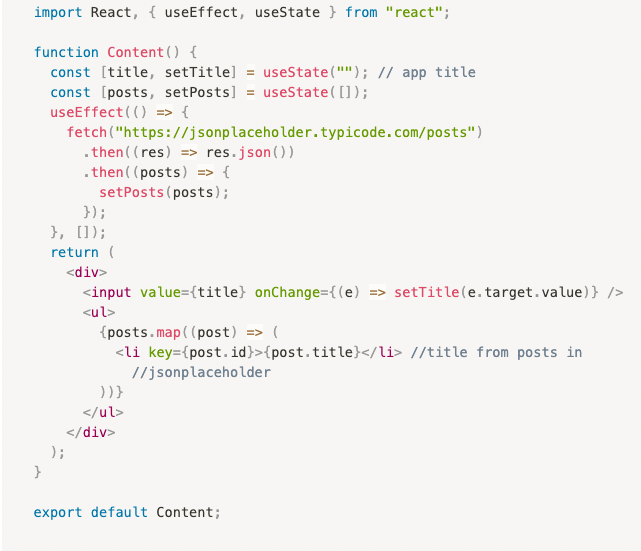
If we call api without putting it in the useEffect, every time component is re-render api will call again, and this is not good, we just want to call api one time
=> without [] in the useEffect, the view on the browser looks fine, and we still see the content what we render to ui, but behind the application network, api is called constantly like a infinity loop => bad
- Another example of case 2, we use the useEffect with Listen DOM EVENTS
- What is DOM Events? HTML DOM Events allow JavaScript to register different event handlers on elements in an HTML document. Events are normally used in combination with functions, and the function will not be executed before the event occurs (such as when a user clicks a button).
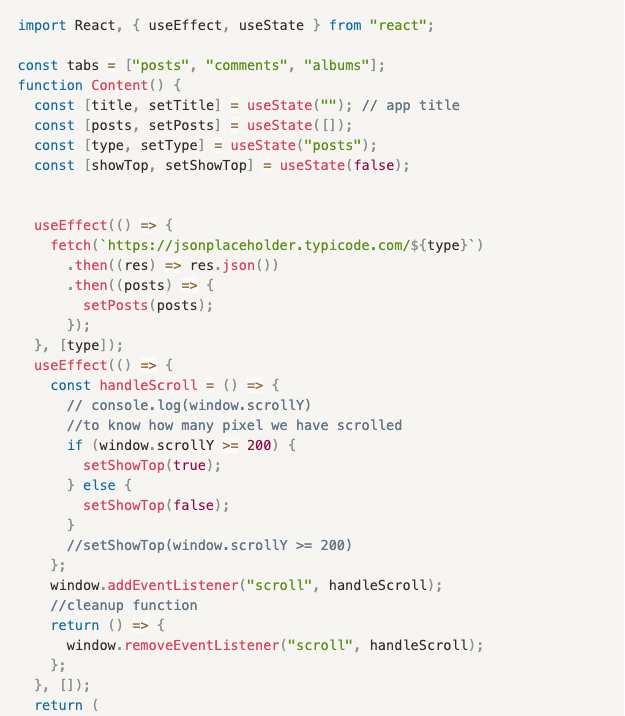
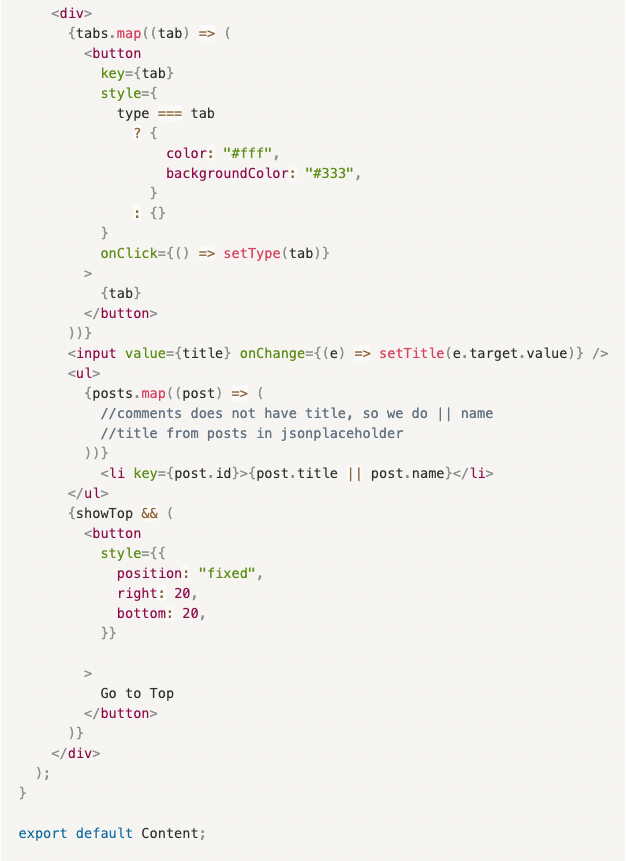
When we do addEventListener to window, even component unmounted, the event is still listening, when we mount component again, browser will remember the old event bind with the unmounted component, and this leads to error: Can’t perform a React state update on an unmounted component. This is a no-op, but it indicates a memory leak in your application.
So we always have to:
return () => {
window.removeEventListener("scroll", handleScroll);
};
Case 3:
Our component renders multiple elements, so to ask react to only call callback if a specific variable is changed, we use case 3. In this example, callback is called whenever ‘type’ changes, and if we change value of ‘title’ in input, callback is not called.
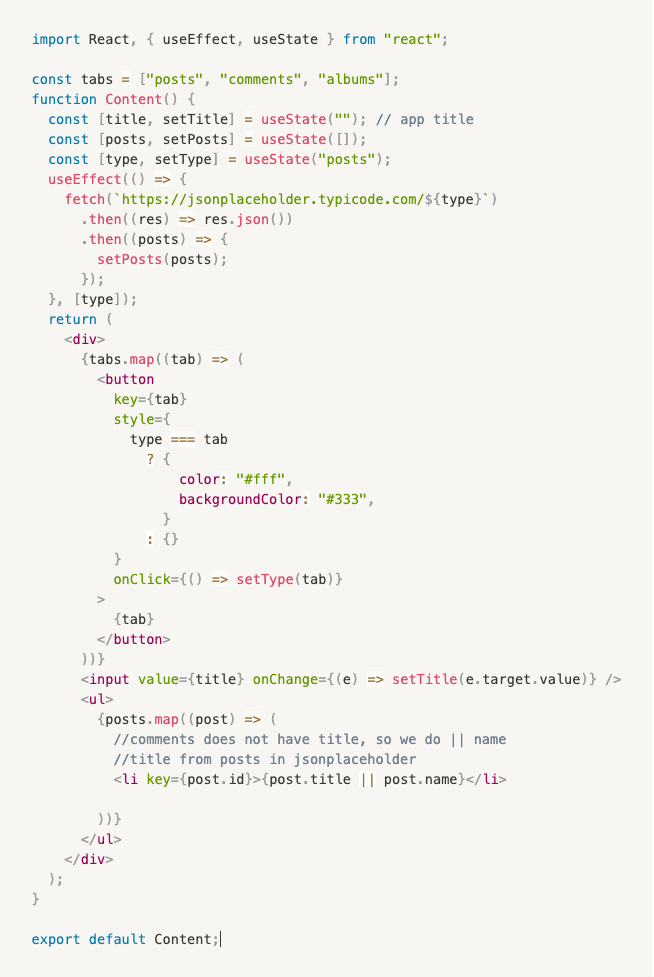
In conclusion, the useEffect hook is a powerful tool in React that allows developers to manage side effects in a functional and efficient manner. By separating concerns and abstracting away state management, useEffect allows for a cleaner and more organized codebase, making it easier to maintain and improve upon in the future. understanding its proper use and implementation is essential for creating dynamic and responsive web applications.
Thank you for taking the time to read this article. It is my hope that the information presented was helpful and provided valuable insights. If you have any questions or comments, feel free to reach out. Thank you again for your time and consideration.
Wonderful! I’m learning a lot